Last updated on August 16, 2024 pm
这次课程创建了一个小组件,
下载了dev tools
一、代码
(1)App.js
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69
| import { useState } from "react";
const messages = [ "Learn React ⚛️", "Apply for jobs 💼", "Invest your new income 🤑", ];
export default function App() { const [step, setStep] = useState(1); const [isOpen, setIsOpen] = useState(true);
function handlePrevious() { if (step > 1) setStep((s) => s - 1); }
function handleNext() { if (step < 3) setStep((s) => s + 1); }
return ( <> <button className="close" onClick={() => setIsOpen((is) => !is)}> × </button> {isOpen && ( <div className="steps"> <div className="numbers"> <div className={`${step >= 1 ? "active" : ""}`}>1</div> <div className={`${step >= 2 ? "active" : ""}`}>2</div> <div className={`${step === 3 ? "active" : ""}`}>3</div> </div>
<StepMessage step={step}>{messages[step - 1]}</StepMessage>
<div className="buttons"> <Button bgColor="#7950f2" textColor="#fff" onClick={handlePrevious}> <span>👈</span>Previous </Button> <Button bgColor="#7950f2" textColor="#fff" onClick={handleNext}> Next<span>👉</span> </Button> </div> </div> )} </> ); }
function StepMessage({ step, children }) { return ( <div className="message"> <h3>Step {step}</h3> {children} </div> ); }
function Button({ textColor, bgColor, onClick, children }) { return ( <button style={{ backgroundColor: bgColor, color: textColor }} onClick={onClick} > {children} </button> ); }
|
(2)index.css
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92
| * { box-sizing: border-box; }
body { font-family: sans-serif; color: #333; }
.steps { width: 600px; background-color: #f7f7f7; border-radius: 7px; padding: 25px 100px; margin: 100px auto; }
.numbers { display: flex; justify-content: space-between; }
.numbers > div { height: 40px; aspect-ratio: 1; background-color: #e7e7e7; border-radius: 50%; display: flex; align-items: center; justify-content: center; font-size: 18px; }
.numbers .active { background-color: #7950f2; color: #fff; }
.message { text-align: center; font-size: 20px; margin: 40px 0; font-weight: bold;
display: flex; flex-direction: column; align-items: center; }
.buttons { display: flex; justify-content: space-between; }
.buttons button { border: none; cursor: pointer; padding: 10px 15px; border-radius: 100px; font-size: 14px; font-weight: bold;
display: flex; align-items: center; gap: 10px; }
.buttons button span { font-size: 16px; line-height: 1; }
h3 { margin: 0; text-transform: uppercase; }
.close { position: absolute; top: 16px; right: 16px; border: none; background: none; cursor: pointer; font-size: 40px; color: inherit; }
.close:hover { color: #7950f2; }
|
二、重点回顾
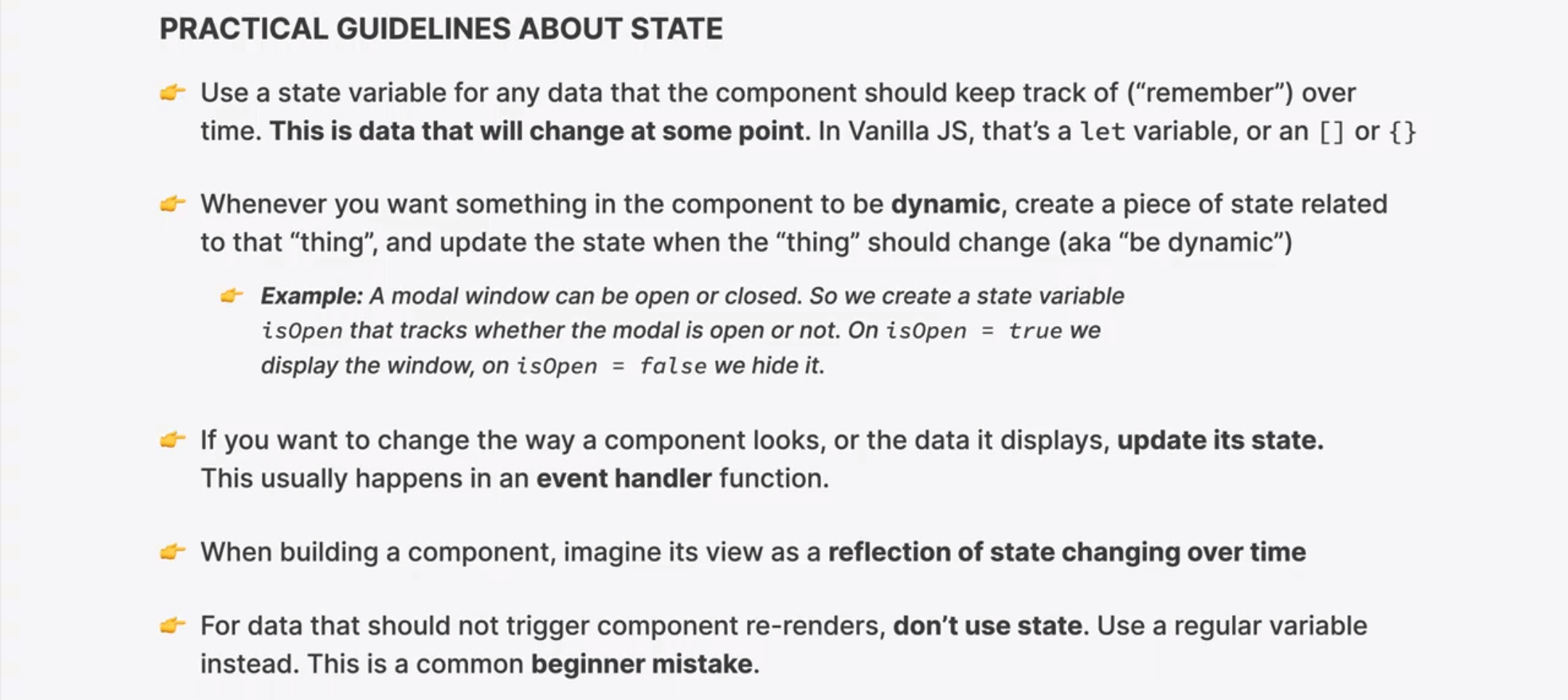
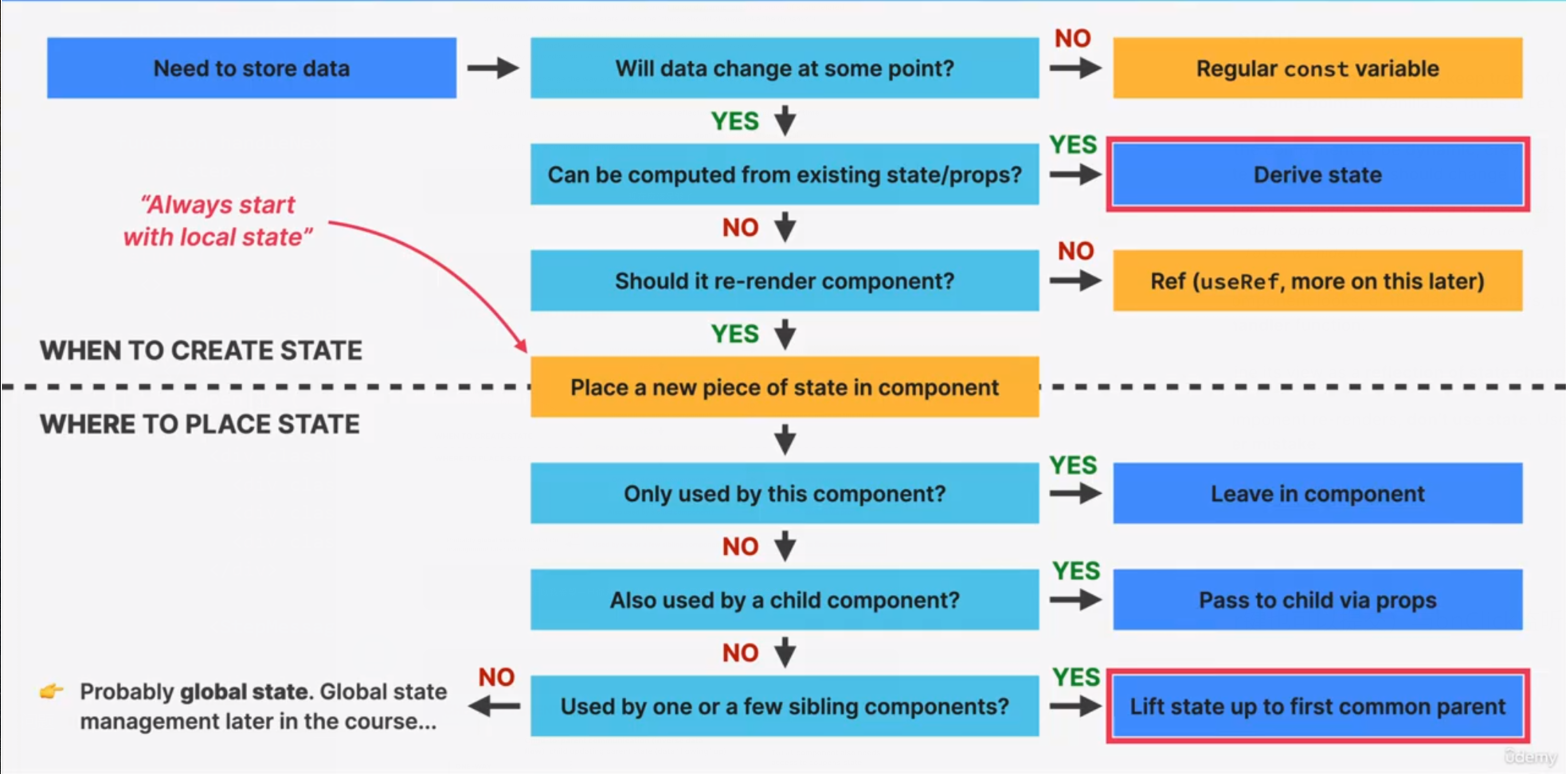
状态的应用(非常之重要)
回调函数
html表单:onSubmit(按下Enter键也可以提交)与onClick监听事件的不同
受控元素
onClick()监控
状态提升(到最近公共祖先)
重用组件、children传递
react课程4-compoment
http://example.com/2024/08/13/react课程4-compoment/