react课程15-使用Tailwind设计项目界面
Last updated on September 18, 2024 pm
本次课程学习Tailwind,并使用它来设计fast pizza的界面🫡。
⭕https://tailwindcss.com/docs/installation(官网)
⭕https://tailwind.org.cn/docs/installation(中文网址)
按照步骤去使用
但是在css文件中会报错:Unknown at rule @tailwindcss(unknownAtRules)
解决方法是打开setting.json文件,在里面添加:
1 |
|
(思考了一个小时的问题😇,世界上还是聪明的人多哈)
⭕安装Tailwind CSS拓展
⭕https://github.com/tailwindlabs/prettier-plugin-tailwindcss?tab=readme-ov-file
安装prettier-plugin-tailwindcss插件:
npm install -D prettier prettier-plugin-tailwindcss
然后进行配置,新建prettier.config.cjs文件,在里面配置:
1 |
|
这样可以自动排序类名
(我怎么配置了两个小时……我真的流泪了。。。。)
🆒https://css-tricks.com/emoji-as-a-favicon/
一、学习初步使用
(1)color
在tailwind的官网中搜索text color、background color查找具体配置说明
index.html中也可以进行配置,来设置全局style
(2)Text
font size(大小)、font weight(加粗)、uppercase(大写)、letter spacing(间距)
(3)space
margin(外边距)、padding(内边距)、border(边框)
space
:主要用于 Tailwind CSS 中,用于设置多个子元素之间的间距,不改变第一个和最后一个子元素的外边距。例如,space-x-4
会在每个子元素之间增加 4 的水平间距,但不会影响最外层的左右间距。
display:
- **
block
**:用来定义内容需要占满一整行的元素(如段落、容器等)。 - **
inline
**:适合内容较短、需要与其他元素同一行显示的情况(如文字、链接等)。 - **
inline-block
**:用于需要水平排列的元素,并且每个元素有独立的宽高控制(如导航栏中的按钮)。 - **
flex
**:用来创建灵活的、响应式的布局,子元素可以自动调整排列。 - **
grid
**:适合构建复杂的、结构化的页面布局,子元素可以按照网格规则自动排布。 - **
none
**:用于隐藏不需要显示的元素。
(4)断点
breakpoints
(断点)用于实现响应式设计。断点定义了在不同的屏幕宽度范围内,如何应用不同的样式规则。这使得可以根据设备的屏幕大小调整页面布局和样式,以提供更好的用户体验。
sm
: 小屏幕(手机,最小宽度 640px)md
: 中等屏幕(平板,最小宽度 768px)lg
: 大屏幕(桌面,最小宽度 1024px)xl
: 超大屏幕(大桌面,最小宽度 1280px)2xl
: 超超大屏幕(超大桌面,最小宽度 1536px)
eg:
1 |
|
如果 Tailwind 的默认断点不符合你的需求,可以在 tailwind.config.js
文件中进行自定义配置。例如:
1 |
|
(5)Flexbox
items-center : 用于在 Flexbox 容器的交叉轴(垂直轴)上将所有子元素居中对齐。
justify-between :用于在 Flexbox 容器的主轴(水平轴)上,将所有子元素之间的空间均匀分配。
(6)Grid
grid h-screen grid-rows-[auto_1fr_auto]
(7)Form element
className="w-28 rounded-full bg-yellow-100 px-4 py-2 text-sm transition-all duration-300 placeholder:text-stone-400 focus:outline-none focus:ring focus:ring-yellow-600 focus:ring-opacity-50 sm:w-64 sm:focus:w-72"
(8)重用
1、在css中编写类名
此时在className=“input”就可以运用这个类名
1 |
|
2、在React中新建组件
eg:这里创建了一个既可以被当作button又可以被当作Link的可重用组件
1 |
|
(9)配置
可以查看默认配置
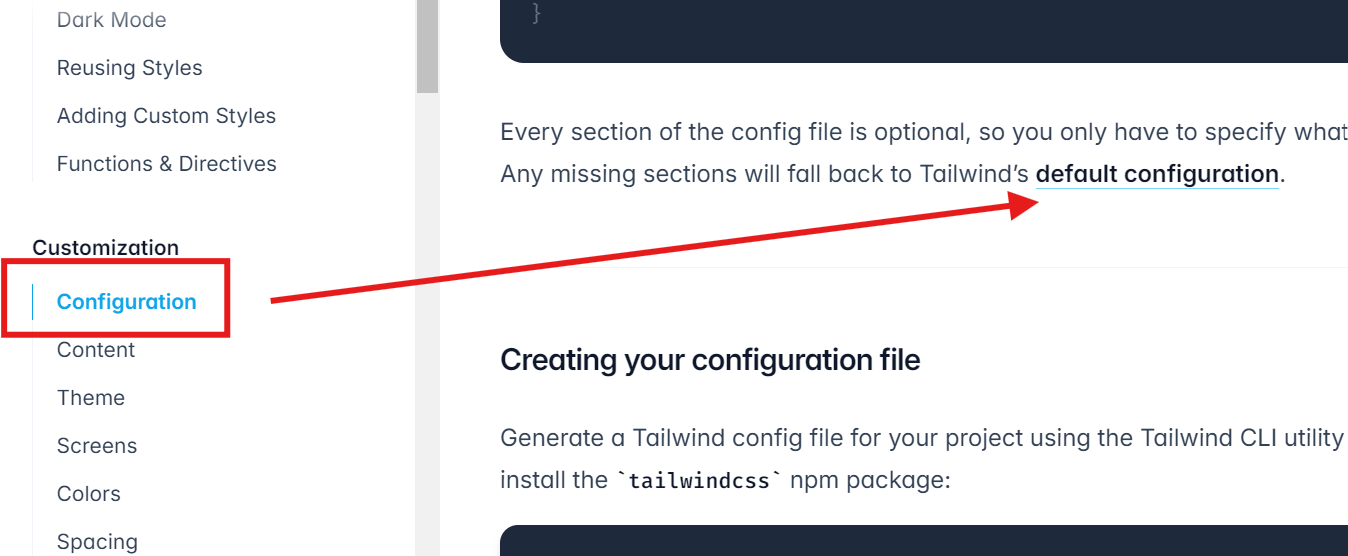
修改默认配置:在tailwind.config.js
中:
(按照默认配置文件的格式)
1 |
|
(10)type
Button组件:
接收type props,并使用styles来应用不同类名
1 |
|