Last updated on November 1, 2024 am
总结一下数组的常用方法
参考CSDN:青松pine
简单属性:
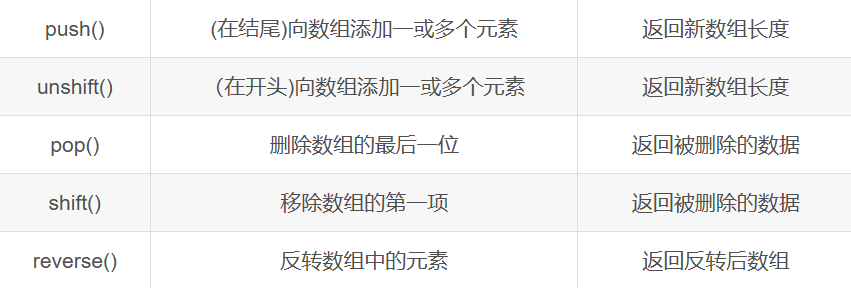
一、构建数组及其长度
数组字面量方法:const fruits = ['apple', 'banana', 'cherry'];
Array构造函数:
new Array(1, 2, 3)
( [ 1 , 2 , 3 ] )
new Array(5)
(长度为5的空数组)
String.prototype.split()
:const fruits3 = "Apple, Banana".split(", ");
(1)Array.from()
从可迭代或类数组对象创建一个新的浅拷贝的数组实例。
1、从字符串构建
1 2
| Array.from("foo"); // [ "f", "o", "o" ]
|
2、从Set构建
1 2 3
| const set = new Set(["foo", "bar", "baz", "foo"]); Array.from(set);
|
3、从Map构建
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| const map = new Map([ [1, 2], [2, 4], [4, 8], ]); Array.from(map);
const mapper = new Map([ ["1", "a"], ["2", "b"], ]); Array.from(mapper.values());
Array.from(mapper.keys());
|
4、从类对象构建
1 2 3 4 5
| function f() { return Array.from(arguments); } f(1, 2, 3);
|
5、箭头函数和 Array.from()
1 2 3 4 5 6 7
| Array.from([1, 2, 3], (x) => x + x);
Array.from({ length: 5 }, (v, i) => i);
|
(2)Array.of()
比较独特的是使用构造函数创建的时候,只写一个数字会创建长度为它的空数组,而这个方法只会直接创建含有那个数字的数组。
1 2 3
| Array.of(); Array.of(1,2,3,4); Array.of(5);
|
(3)长度
🐣Array.length是一个小于2^32的非负整数
数组对象会观察 length
属性,并自动将 length
值与数组的内容同步。这意味着:
- 设置
length
小于当前长度的值将会截断数组——超过新 length
的元素将被删除。
- 设置超过当前
length
的任何数组索引(小于 232 的非负整数)将会扩展数组——length
属性增加以反映新的最高索引。
- 将
length
设置为无效值(例如负数或非整数)会引发 RangeError
异常。
当 length
被设置为比当前长度更大的值时,数组通过添加空槽来扩展,而不是实际的 undefined
值。空槽与数组方法有一些特殊的交互作用;详见数组方法和空槽。
二、按顺序排列的数组方法
(1)at()
at() 方法接收一个整数值并返回该索引对应的元素,允许正数和负数。负整数从数组中的最后一个元素开始倒数(即index+array.length位置)
返回数组中与给定索引匹配的元素。如果 index < -array.length
或 index >= array.length
,则总是返回 undefined
,而不会尝试访问相应的属性。
🦃非数组对象也可以调用:
1 2 3 4 5 6
| const arrayLike = { length: 2, 0: "a", 1: "b", }; console.log(Array.prototype.at.call(arrayLike, -1));
|
(2)concat()
concat
方法创建一个新数组。该数组将首先由调用它的对象中的元素填充
1 2 3 4 5 6 7 8
| const num1 = [1, 2, 3];const num2 = [4, 5, 6];const num3 = [7, 8, 9]; const numbers = num1.concat(num2, num3);
const letters = ["a", "b", "c"]; const alphaNumeric = letters.concat(1, [2, 3]);
const num1 = [[1]];const num2 = [2, [3]]; const numbers = num1.concat(num2);
|
在稀疏数组上调用,结果的数组也是稀疏的。
(3)every
every()
方法测试一个数组内的所有元素是否都能通过指定函数的测试。它返回一个布尔值。(是一种迭代方法,为数组中除空槽外所有元素调用callbackFn函数,直到返回一个假值)
示例:判断一个数组是否是另一个数组的子集
1
| const isSubset=(array1,array2)=>array2.every(el=>array1.includes(el));
|
(4)fill()(是修改方法)
- fill(value)
- fill(value, start)
- fill(value, start, end) (区间左闭右开)
1 2 3 4 5 6 7 8 9 10
| const array1 = [1, 2, 3, 4];
console.log(array1.fill(0, 2, 4));
console.log(array1.fill(5, 1));
console.log(array1.fill(6));
|
- 如果
value
是个对象,那么数组的每一项都会引用这个元素。
- 负索引从数组的末端开始计算
- 超过数组范围的索引填充会被忽略
- 如果经标准化后,
end
的位置在 start
之前或之上,没有索引被填充。
- 会填充稀疏数组的空槽
🦫如果 value
是个对象,那么数组的每一项都会引用这个元素。
1 2 3
| const arr = Array(3).fill({}); arr[0].hi = "hi";
|
(4)filter()
传入一个callback函数,只有满足条件的才会被留下。
⚠️在callback中修改数组是真的会修改原数组。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33
| let words = ["spray", "limit", "exuberant", "destruction", "elite", "present"];
const modifiedWords = words.filter((word, index, arr) => { arr[index + 1] += " extra"; return word.length < 6; });
console.log(modifiedWords);
words = ["spray", "limit", "exuberant", "destruction", "elite", "present"]; const appendedWords = words.filter((word, index, arr) => { arr.push("new"); return word.length < 6; });
console.log(appendedWords);
words = ["spray", "limit", "exuberant", "destruction", "elite", "present"]; const deleteWords = words.filter((word, index, arr) => { arr.pop(); return word.length < 6; });
console.log(deleteWords);
|
(5)find
find方法返回数组中满足提供测试函数的第一个元素的值。(没有找到则返回undifined)